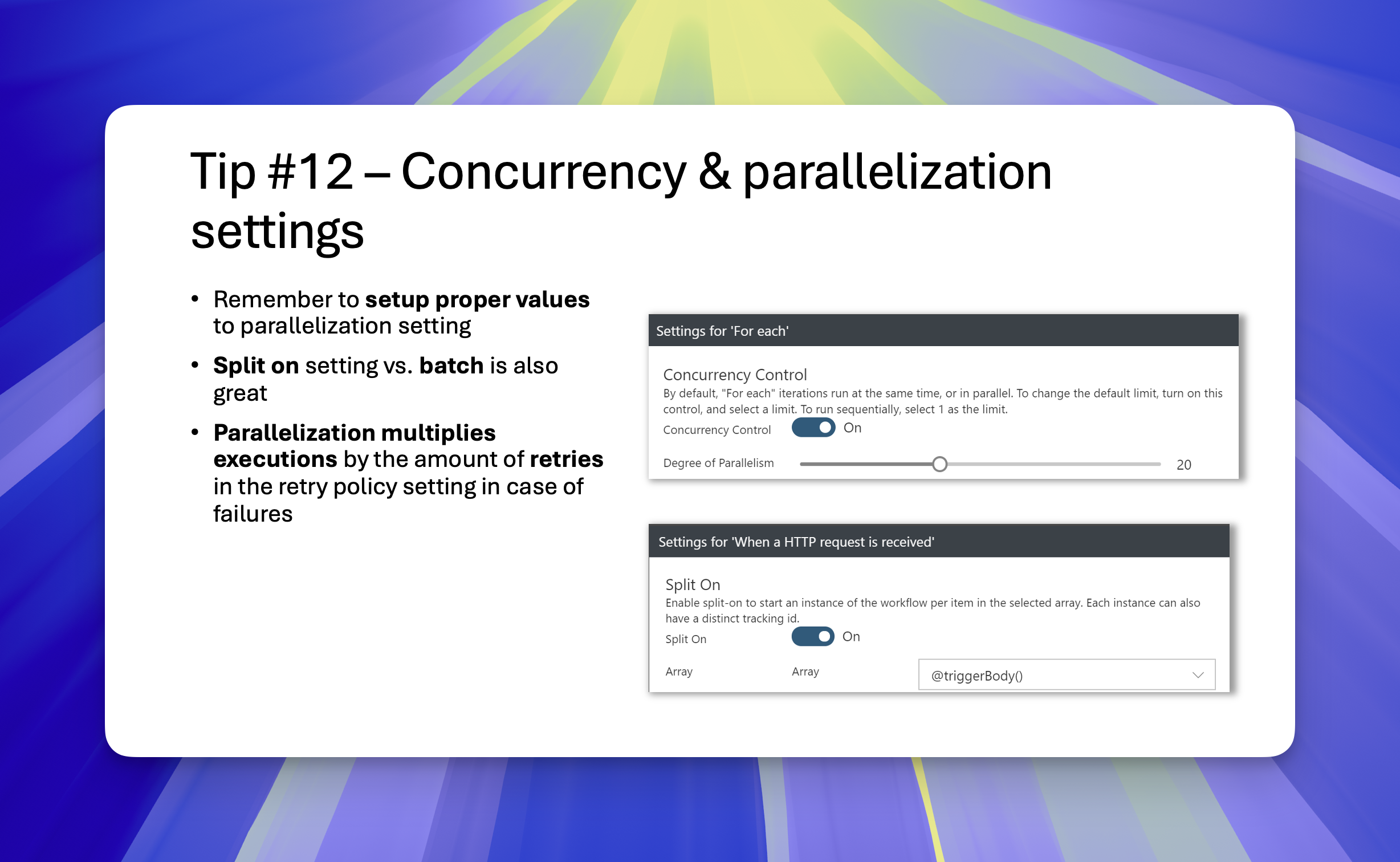
Highlight
It’s a small thing, but somehow many people forget about this.
Intro
I can’t count the amount of times that I’ve had to look into a case of
- Expensive logic apps
- Long running logic apps
- Failing logic apps
- Random cases of overwritten variables
- and more…
Only to find out it was either forgotten parallelization or concurrency behaviors
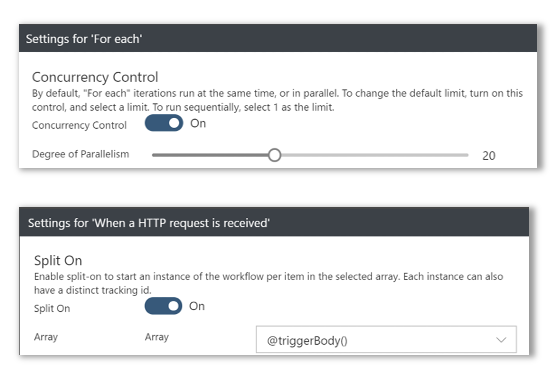
Parallelization
Most logic app actions that iterate have support for parallelization. It’s basically a setting of how many executions of the iteration to do at the same time. This one can be confusing for those coming from other programming languages where for-each loops are sequential by default. If you want to have the same behavior set parallelization setting to 1.
Old logic app designer showed this when clicking “…” three dots, and selecting settings option. New logic app designer, is much cleaner and this setting can be found in “settings” tab.
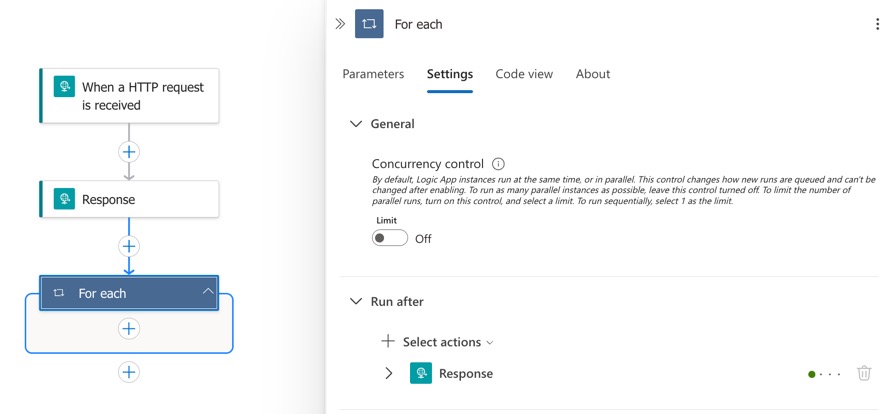
Note that the default is set to “off” this means logic app will try to parallelize this as much as possible. But that max is 50 anyways, so don’t worry about it.
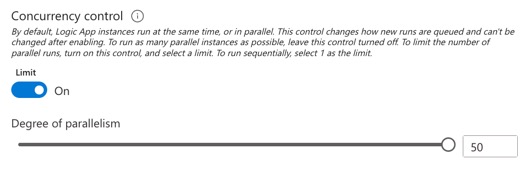
Why should I care?
Let’s make an example. If you use ‘set variable’ or similar action which overrides existing variable or state of any object, if you parallelize you will run into racing conditions. Racing condition basically means, if you run 50 parallel threads, each tries to set a variable, all of them override it, and following actions which use these variables will act oddly which will be very hard to debug.
For example let’s assume our logic app is a simple one with two actions
- Loop through users ‘Adam’ and ‘Tom’
- Set variable ‘UserName’ to current username to process
- Set ‘out of office’ setting to that user (from variable UserName)
And then execute them in parallel
- Start loop for Adam and Tom - 2 parallel executions
- Loop #1 sets variable ‘UserName’ to ‘Adam’
- Loop #2 sets variable ‘UserName’ to ‘Tom’
- Loop #1 uses ‘UserName’ (currently set to ‘Tom’) variable to set out of office. Hence Tom has now out of office message.
- Loop #2 uses ‘UserName’ (currently set to ‘Tom’) variable to set out of office. This either fails because Tom already has out of office set, or just does nothing.
Problem is fairly obvious, user Adam did not get his out of office setting becaus loop 2 changed variable to Tom before first parallel loop could do anything with it.
So yea, parallelization is important. Especially with scoped variables.
But other scenarios where parallelization is important is
- Long running workflows - technically you want to parallelize, just use actions that are scoped like ParseJson or ComposeJson which can act like Initialize or Set Variable
- Calling external APIs with rate limits - too many calls with start throwing errors, which in conjution with retry policies will cause major slow down of your logic app and potentially significantly raise the cost (read more in retry policies section)
Parallelization on triggers
Did you know that you can greatly increase parallelization of you logic apps if you use ‘SplitOn’ setting on your triggers? Many triggers do that by default, but if you haven’t explored it make sure you do.
For example, imagine you have this JSON as paylod for you logic app HTTP request trigger
{
items: [
{
name: "Adam"
},
{
name: "Tom"
},
{
name: "Jake"
},
{
name: "Jane"
}
]
}
You can use SplitOn on your trigger using expression RequestBody().items
which execute 4 entire new logic app workflows incread 1 workflow with 4 items as payload. Each instance would get instead a payload like this
Logic App Instance #1
{
name: "Adam"
}
Logic App Instance #2
{
name: "Tom"
}
Logic App Instance #3
{
name: "Jake"
}
Logic App Instance #4
{
name: "Jane"
}
This is very neat and can be used to greatly increase parallelization of your logic apps.
When not to
Just remember to not parallelize when you need to ensure order or processing everything in a single transaction. Always use common sense for your use-case.
Summary
I think we can all agree that while this is fairly straightforward, I really can’t count the amount of times I’ve seen someone struggle with logic apps at scale because of having these two core concepts wrong.
Now that you know this you can take your logic app game to the next levels.