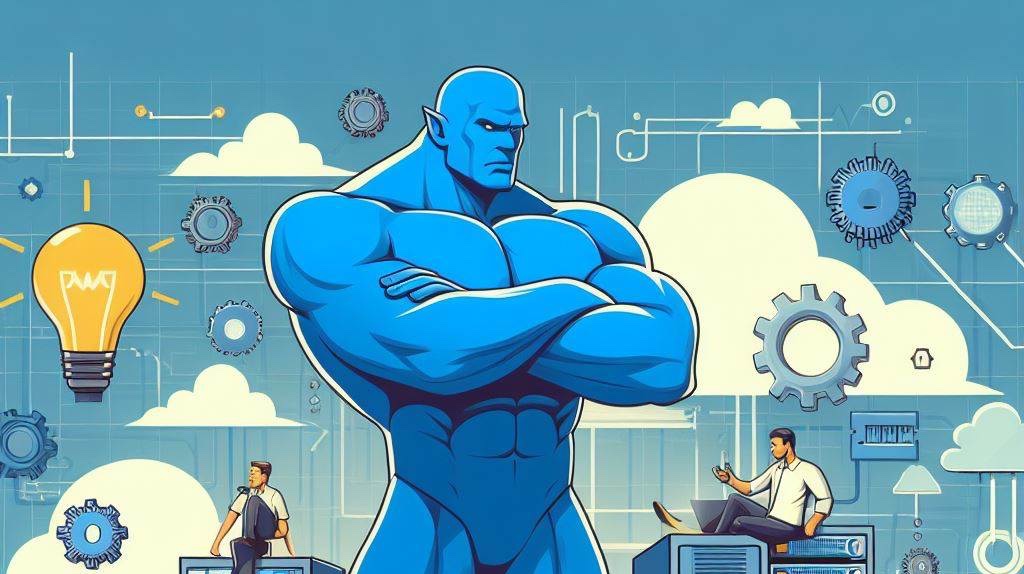
Highlight
How to update (merge) your app setting without overriding them using Azure Bicep? Let me show you :)
It’s actually easier than you think.
Module file for settings
You will need a separate file with app settings configuration from the app service module.
App Service Config Module
param appServiceName string
param appSettings object
param currentAppSettings object
resource webApp 'Microsoft.Web/sites@2022-03-01' existing = {
name: appServiceName
}
resource siteconfig 'Microsoft.Web/sites/config@2022-03-01' = {
parent: webApp
name: 'appsettings'
properties: union(currentAppSettings, appSettings)
}
App Service Module
param logicAppName string
param location string
param logicAppAppInsightsResourceId string
param logicAppVirtualNetworkSubnetId string
param logicAppServicePlanResourceId string
resource logicApp 'Microsoft.Web/sites@2022-09-01' = {
name: logicAppName
location: location
kind: 'functionapp,workflowapp'
tags: union(
resourceGroup().tags,
{ 'hidden-link': logicAppAppInsightsResourceId }
)
properties: {
name: logicAppName
clientAffinityEnabled: false
virtualNetworkSubnetId: logicAppVirtualNetworkSubnetId
publicNetworkAccess: 'Enabled'
httpsOnly: true
serverFarmId: logicAppServicePlanResourceId
siteConfig: {
vnetPrivatePortsCount: 2
}
}
identity: {
type: 'SystemAssigned'
}
}
output logicAppManagedIdentity string = logicApp.identity.principalId
Main File
and then simply execute it from your main bicep file as such (using Logic Apps app service plan as example)
1module logicApp 'modules/logic-apps-standard.bicep' = {
2 name: '${deployment().name}-module-logic-app'
3 params: {
4 location: location
5 logicAppAppInsightsResourceId: logicAppAppInsightsResourceId
6 logicAppName: logicAppName
7 logicAppServicePlanResourceId: logicAppServicePlanResourceId
8 logicAppVirtualNetworkSubnetId: logicAppVirtualNetworkSubnetId
9 }
10 dependsOn: [
11 storageAccount
12 ]
13}
14
15module logicAppSettings 'modules/app-service-app-settings.bicep' = {
16 name: '${deployment().name}-module-logic-app-settings'
17 params: {
18 appServiceName: logicAppName
19 currentAppSettings: list(resourceId('Microsoft.Web/sites/config', logicAppName, 'appsettings'), '2022-03-01').properties
20 appSettings: {
21 FUNCTIONS_EXTENSION_VERSION: '~4'
22 FUNCTIONS_WORKER_RUNTIME: 'node'
23 WEBSITE_NODE_DEFAULT_VERSION: '~18'
24 APPLICATIONINSIGHTS_CONNECTION_STRING: reference(resourceId(logicAppInsightsResourceGroupName, 'Microsoft.Insights/components', logicAppInsightsName), '2020-02-02').ConnectionString
25 AzureWebJobsStorage: 'DefaultEndpointsProtocol=https;AccountName=${storageAccountName};AccountKey=${listKeys(resourceId('Microsoft.Storage/storageAccounts', storageAccountName), '2023-01-01').keys[0].value};EndpointSuffix=core.windows.net'
26 WEBSITE_CONTENTAZUREFILECONNECTIONSTRING: 'DefaultEndpointsProtocol=https;AccountName=${storageAccountName};AccountKey=${listKeys(resourceId('Microsoft.Storage/storageAccounts', storageAccountName), '2023-01-01').keys[0].value};EndpointSuffix=core.windows.net'
27 WEBSITE_CONTENTSHARE: 'amdemoapp'
28 AzureFunctionsJobHost__extensionBundle__id: 'Microsoft.Azure.Functions.ExtensionBundle.Workflows'
29 AzureFunctionsJobHost__extensionBundle__version: '[1.*, 2.0.0)'
30 APP_KIND: 'workflowApp'
31 }
32 }
33 dependsOn: [
34 logicApp
35 ]
36}
And now the flow looks like this
- On first deployment
- App is created, no settings are merged as none are present
- On subsequent deployments
- If new settings are present pull them into bicep (line 19)
- Add new settings or override existing (line 20-31)
- Module merges inputs from 2.1 and 2.2 and updates app service with union of both
Enjoy!