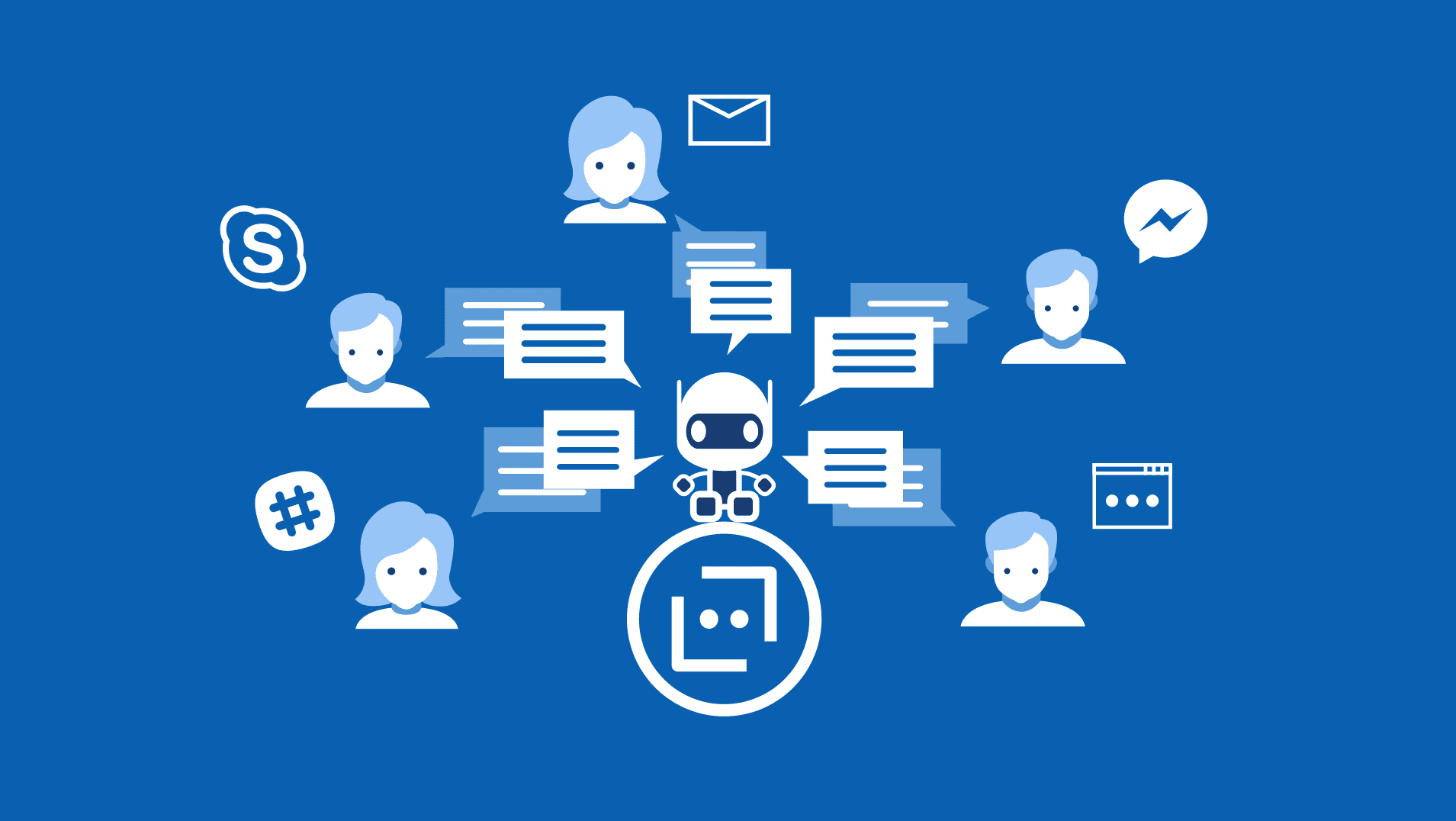
Highlight
Microsoft Bot Framework with conjunction with Azure Bot Service provides the platform for quick bot development. Learn what are chat bots for and how to leverage those tools to deliver basic bots in just minutes.
In recent years increased availability of internet across the world and evolution of mobile devices popularized messaging applications which became most common way to communicate in private life as well as everyday business. It is no surprise that leveraging those communication channels has huge potential in any business.
What are chat bots?
Chat bots are applications whose main role is extension of usually already existing applications and therefore it is often said that they are application interfaces. While a developer can build full-fledged application purely within chat bot app it is not what chat bots are for.
Bots can handle multitude of business scenarios, few examples are
- Level 0/1 support roles responding to basic user queries.
- Granting access to resources and websites.
- Informing of events.
- Prompting for approvals and other actions.
- Allowing control over systems. Fantastic example is Azure Bot in bot directory.
- Knowledge base for wiki or FAQ
Often people wonder if chat bots are win it all application. They are not. But they have fantastic set of uses and they are best for enhancing service quality not completly removing human interaction from them. Best scenario for bots is to handle 50-80% of common cases and redirect users to human for cases that bot is not suited to do. While it’s possible to code those cases too it’s often not worth the cost.
How are chat bots built?
Building bots with use of Microsoft stack usually consists of two main components
Those are connected as follows
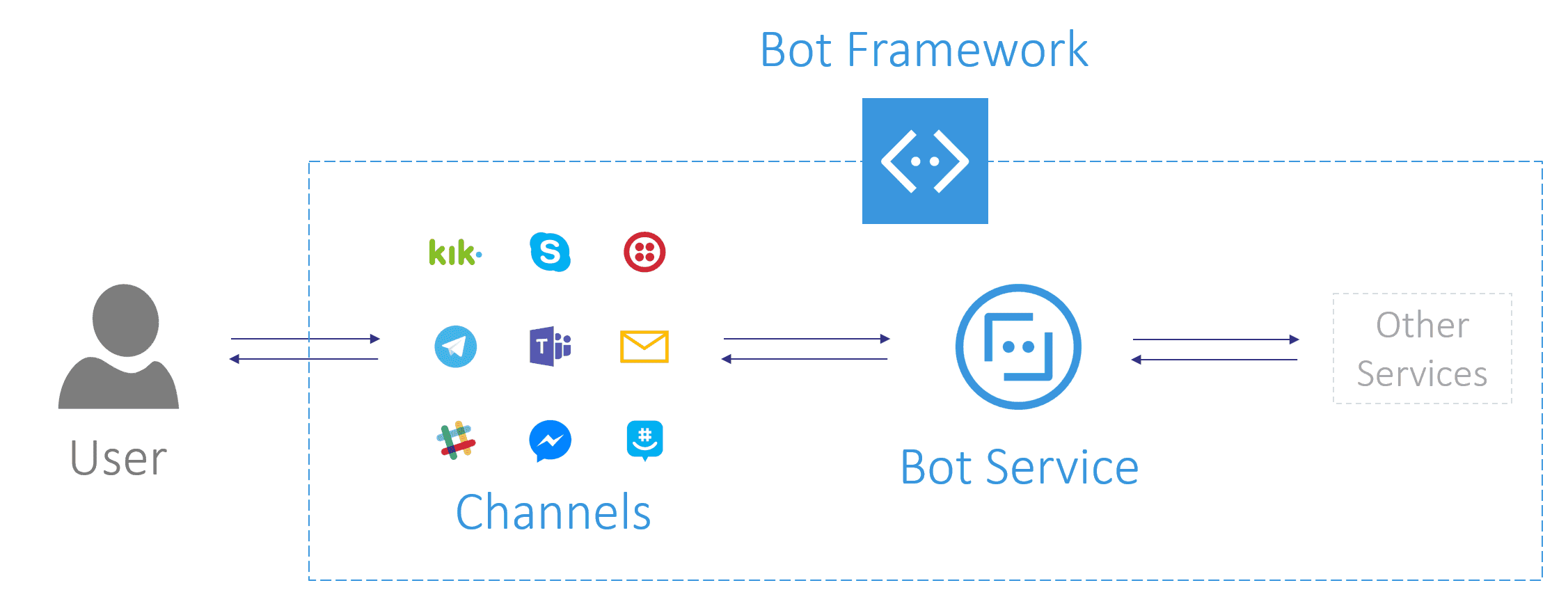
Microsoft Bot Framework
Open source SDK and platform delivered by Microsoft for rapid bot development.
Platform consists of several components
- Bot Builder SDK
- Open Source library written with .NET and Node.js available at github for anyone to explore and contribute.
- REST API for those who want to integrate it from different languages and platforms.
- Bot Emulator for testing bots locally and remotely without a need for any channel. It works great with Ngrok tunneling tool for remote connectivity
- Bot Connectors (channels) which are API proxies delivered and maintained by Microsoft which connect to existing services and proxy/format messages between the messaging platform (client origin) and bot service.
- Two enabled by default which are SKype and Web Chat
- And 12 more to configure which include Bing, Cortana, Direct Line, Email, Messenger, GroupMe, Kik, Microsoft Teams, Skype for Business, Slack, Telegram and Twillio SMS
- Registration and management portal (fully integrated with Azure Bot Service) for maintaining directory of bot applications.
- Bot Catalog - bot directory is great place to find real examples of useful bots that are ready to be implemented in any company.
Azure Bot Service
Bot Service is specialized version of Azure App Service with full web IDE (just like Azure Functions) as well as basic tooling for bot development and management. Key features of this service are
- IDE for bot development
- Channel configuration page
- Application settings and web configuration
- Chat testing tools and logging/analytics
Creation of a chat bot
In this article a basic form bot will be built which will serve users with a questionnaire about their favorite mobile device and color. Nothing complicated but it gives a decent idea of how bots operate and how developer can create simple bot in just minutes.
Instead of reading you can watch!
Or just follow the steps
- Log into Azure Portal
- Find Azure Bot Service in Marketplace
- Create Bot Service
- Fill in the data.
- Consumption Plan will Functions pricing model (pay per execution). Preferred for most bots.
- App Service Plan will use App Service (pay for reserved capacity). Preferred if using Free Tier for learning purposes.
- Click create button redirects to bot wizard where C# should be set language and Form as a bot template.
- Microsoft App Registration page where application must be first registered.
- Once bot is created developer is transferred back to Azure Development portal and after hitting Create button the bot is provisioned. Provisioning takes between 2 and 5 minutes and during that time template sets up Bot Framework application, storage account for the code and logic, bot service application and application insights.
- Test the bot with IDE chat control
Additionally, developer can set up Bot Emulator for testing which takes just minutes.
- Download Emulator from Bot Framework website
- Download Ngrok tunneling tool for remote connectivity and extract it at convenient location
- Run Bot Emulator
- Open App Settings
- Provide path to ngrok.exe where it was previously extracted
- Click on the ‘Enter your endpoint URL’ text bot in emulator and provide bot details
- Get messaging endpoint to chatbot by going to Azure Portal IDE and selecting Settings Tab. Scroll down to Configuration section and copy paste the link into emulator
- Emulator will prompt for Microsoft App ID and Password, to get App ID again scroll down in app settings tab
- To get password click on Open button in Application Settings section and scroll down to field called MicrosoftAppPassword and copy its value
- Click Connect
- Test the bot
Editing the business logic
Now that the bot is set up and running editing the default logic to mobile device questionnaire is pretty straightforward.
-
Open BasicForm.csx file in the browser IDE and paste in the code
Highlighted lines are the ones that has been changed for purpose of this scenario. So in detailsusing System; using Microsoft.Bot.Builder.FormFlow; public enum MobileOptions { Laptop = 1, Smartphone, Tablet }; public enum ColorOptions { Black = 1, Silver, Gold, White, Red, Blue, Green }; [Serializable] public class BasicForm { [Prompt("Hello! What is your {&}?")] public string FullName { get; set; } [Prompt("Please select your favorite mobile device type {||}")] public MobileOptions MobileDevice { get; set; } [Prompt("Please select your favorite {&} {||}")] public ColorOptions Color { get; set; } public static IForm<BasicForm> BuildForm() { return new FormBuilder<BasicForm>().Build(); } public static IFormDialog<BasicForm> BuildFormDialog(FormOptions options = FormOptions.PromptInStart) { return FormDialog.FromForm(BuildForm, options); } }
-
All string types are translated to simple user prompts for text. This is good when users can’t be provided with options.
-
All enum types are translated to single selection card prompts. This is good when there is set amount of options to choose from.
-
All List<enum> types are translated to multiple selection card prompts. This is good when there is set amount of options to choose from but users should be able to select multiple options. More info about form flows at Bot Framwork Form Flow tutorial
-
Click Save and Test the bot!
That’s it. In just about 10 minutes of work questionnaire bot is done and ready to be deployed to users.
Play around with it!
Now just go and start building chatbots.
Source Code